JavaScript is the most fun language I have ever played with. Today, I’m a full-stack web developer working in London as part of a fab team. So, I have brilliant and experienced people around me to guide me. However, two years ago, when I first decided to learn JavaScript, I remember being very confused about where to start. There are way too many frameworks and courses to choose from and I didn’t know how to make those choices. In this article, I make some of those difficult choices for you and help you get started with JavaScript. The goal is to help you rapidly reach a point where you know enough to figure out your own way forward. I hope you find it useful.
Why JavaScript - the one language to rule them all
There are so many languages to choose from. Why choose JavaScript?
Well, if this is your first time learning a programming language, Python may be a better one to start with. You can read more about Python in my post on getting started with Python. Web development involves understanding not just JavaScript, but also HTML and CSS and having a basic understanding of how the Internet and the browser works. It can intimidate you and distract you from understanding the fundamentals of programming. JavaScript also has various quirks that can confuse beginners.
Once you have developed a good foundation on Python, you can build on it with JavaScript. According to the 2019 stackoverflow survey, JavaScript is the most popular technology beating both Python and Java. JavaScript/web development is especially satisfying because:
- It enables you to create modern-looking user interfaces.
- You can easily share it with the whole world via the Internet.
I found Python very lacking in both of the above aspects. Furthermore, if making web apps is your only motivation for learning programming, JavaScript, or a flavour of it, is pretty much your only option!
JavaScript is the lingua franca of the web
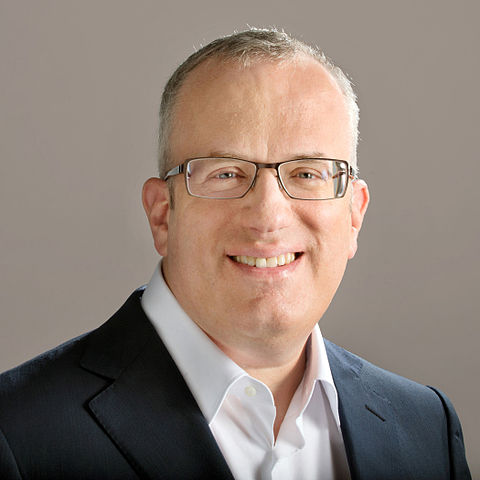
Brendan Eich - the creator of JavaScript
Brendan Eich created JavaScript in 1995 during his time at Netscape Communications. It was famously made under very tight deadlines to meet the demand for a script that allowed interactivity in the web browser. The rushed work resulted in plenty of design quirks that we have to live with today. See this rant for example.
Despite this, its flexibility is a strength that has allowed the JavaScript ecosystem to evolve drastically to suit the insatiable demand of web developers and consumers. Those of us who love JavaScript accept and love the flaws in the language. Some may even call it true love. :P
I highly recommend watching the following video about the evolution of JavaScript. A brief history of JavaScript is also a good read.
React is the popular kid right now
Writing a website with vanilla JavaScript is hard work. Instead, we normally use frameworks that reduce the burden on the developer. Frameworks handle a lot of the more menial work behind the scenes and allow you to focus on the meat of your application.
There are three prominent front-end frameworks today: React, Angular and Vue.js. As you can see in this 2019 State of JavaScript survey, React, created by facebook, is the most popular one today. React is what I started learning web development with and I’m still using it in my job today. I find it very intuitive to use. I particularly like JSX introduced by React, which allows mixing HTML and JavaScript.
I recommend that you start learning with React, but if this feels like a major life decision to you, as it did for me, try the following video. You can get started with React quickly using the official create-react-app.
Identify a problem to solve using JavaScript
I find it challenging to learn something new without applying it right away. It’s good to have a project in mind as you’re learning. It should not be anything too complicated, but something simple that also motivates you. I can share my first few projects which will hopefully inspire you to find your own pet project.
My first and second apps were elementary things that I made while working at Rolls-Royce. I cannot share them with you as they were internal, but I can describe them to you. Following the fourth app, I got my first job as a web developer and haven’t worked on any personal web apps since.
I had started a lecture series on Python, and I needed a website to record previous lectures. I made a React app whose main feature was a table listing previous lectures with hyperlinks to the lecture resources. It also had a few other pages with instructions for presenters and viewers. Since it was my very first app, it took me something like a week to put together.
There was an old piece of software at work that had an unintuitive UI. The documentation ran for hundreds of pages, and I hated it. Therefore, I created a web app to act as a guide. It had a navigation bar on the left. The user could select a particular workflow using the navigation bar. The right-hand side contained a video demoing the workflow. Beneath the video, written instructions were also provided. I made most of this in one weekend with very little sleep.
I had a major argument with one of my friends. He believed that it was stupid of me not to buy a house and waste my money renting. I build renting vs. buying a house to both put that hypothesis to test and to learn more about React. It was my first significant web app and took several weekends of effort, on and off.
I wanted to make a desktop app using Electron and React and see how difficult it would be. I made a ssh key manager, which to be fair, doesn’t have much practical use. However, I found this very easy to make.

Tools you need
To program in JavaScript, you need some software tools. Here are some good options to start with.
The Chrome browser has an excellent developer console. It’s got a significant slice of the browser market share, over 65%, at the time of writing this article. As you develop your app, you will be checking how it looks and behaves on the browser. The browser is also handy for debugging.
VS Code is a free, open-source editor by Microsoft that’s pure awesomeness - rather uncharacteristic of Microsoft :P. It is the most popular developer environment, according to the 2019 stackoverflow survey.
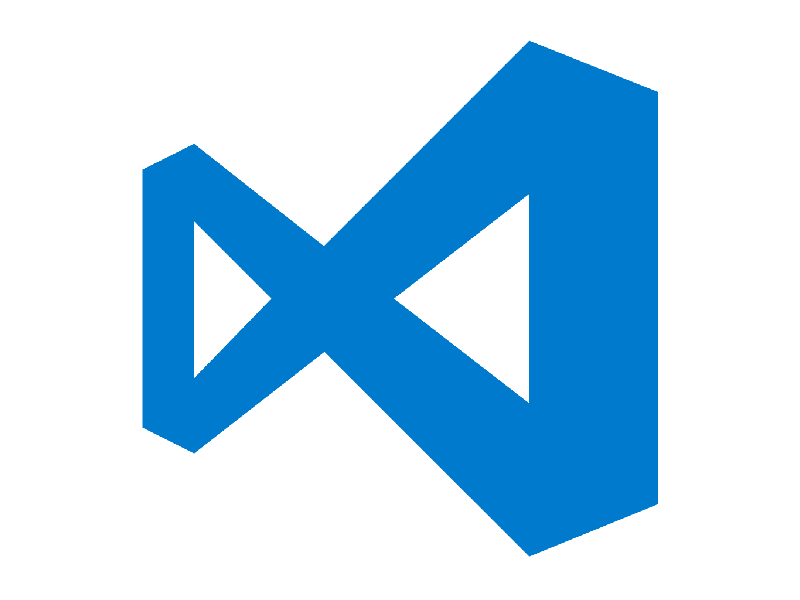
git is a Version Control System (VCS) that lets you keep track of changes in your code. It gives you the freedom of editing your code without worrying about losing it. Being a Distributed Version Control System (DVCS), it also lets you collaborate with other developers to develop a solution to a mutually shared problem. It was created by the living legend, Linus Torvalds, in 2005 for the development of the Linux kernel.
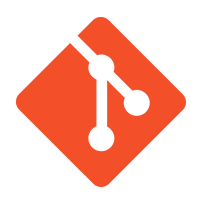
Learning resources
These are the learning resources that I recommend to get started with JavaScript. Notice that a lot of the links below point to FrontendMasters. I use it actively and find it well worth the price.
- Harvard CS50 - This is the best series of lectures I’ve ever seen in my life. I recommend this course to both beginner and intermediate programmers who want to brush up on the basics.
- Introduction to git at Treehouse – This course is a modest introduction to git. It takes some time to build confidence with git, so don’t worry if you find it hard at first. Everyone starts that way.
- Modern React with Redux - This is the course I started learning JavaScript/React with. Stephen Grider is a virtuoso teacher, and I have no doubt you’ll enjoy this course. I love that he uses diagrams extensively to help explain difficult concepts. However, you can find lots of other highly rated courses in Udemy as well.
- Testing React Applications - I enjoy testing so much now, thanks to Kent C. Dodds. He is a very opinionated person with terrific opinions. I hope I’m at least half as cool as this guy one day!
- Mastering Chrome Developer Tools v2 - A lot of beginner developers don’t appreciate how useful the browser dev tools can be. Until I did this course, I was one of them!
- Visual Studio Code Can Do That? - Learn how to use your editor and I promise programming will be so much more fun.
- JavaScript: The Hard Parts, v2 - This course by Will Sentance was a mind-blowing experience for me. It covers callbacks and higher-order functions, closure, asynchronous JavaScript, and object-oriented JavaScript. Some may say this is a bit too much for a beginner, but I strongly disagree. JavaScript is a quirky language, and if you don’t understand what’s under the hood, you could end up writing bad code.
- JavaScript: The New Hard Parts - Another excellent course by Will Sentance. This one covers the new features of JavaScript in ES6+: iterators, generators, promises, and async/await. Async/await was magic to me until Will explained it to me in this course.
Learning goals
These are the topics that I think you need to have become comfortable with as a beginner JavaScript programmer. As you make your way through the learning resources above, these words should start to sound familiar.
- Internet/browser - HTTP, DNS, HTML, CSS, Document Object Model (DOM)
- Basics - operators, arrays and array iteration (map, filter, reduce, find, forEach), objects, conditions, loops, JSON, template literals/strings, array and object destructuring
- More interesting stuff - hoisting, object literals vs constructors, this keyword, scope, functions, fat arrow/lambda/anonymous functions, closures, higher-order functions, classes, prototypes & inheritance, events, error handling
- Asynchronous JS - event loop, call stack, message queue, job queue, promises, async/await
- React - hooks, JSX, React Developer Tools
- Redux - actions, reducers, store, data flow, Redux DevTools
- Dev tools - package management with npm, version control with git, testing with jest and react-testing-library, linting with eslint, formatting with prettier, Chrome debugging, VSCode React debugging
I also use this Front-end Developer roadmap to understand what my learning gaps are.
Other resources
- stack overflow – This is where programmers spend most of their lives (sadly :P). I highly encourage you to ask questions yourself if you cannot find an answer. Not only will you be helping yourself, but also the community out there!
- Udemy - I rely heavily on Udemy to learn about various subjects, and I’m a very happy student. :)
- Github - Github is a place where developers collaborate on code development. Why not check out the source code for one of your favourite packages?
- Observable notebooks are something that I’m very excited about. It lets you write both JavaScript and markdown content in cells. It’s kind of like a Jupyter notebook, but so much better because JavaScript can run in the browser itself and there’s no need for a backend Python server. Not only is it an excellent playground for trying out JavaScript, there are also lots of exciting notebooks out there.
- CodeSandbox is an amazing online IDE for web development. It can be useful when you want to share/collaborate with someone. I don’t use CodeSandbox much because I feel much more at home with VSCode on my Mac.
- Conferences - You don’t know what you don’t know. You don’t have to be an expert in everything, but it’s good to be aware of what’s out there and what’s up and coming. This is why it’s important for programmers to attend conferences. Thankfully, most of these conferences upload their videos to YouTube so that you can watch them for free! It’s okay if you don’t understand everything, as long as you can follow its gist. Over time, this will help you expand your knowledge, and you’ll find yourself capable of following lectures on more advanced topics.
Conclusion
Hopefully, this article has helped you get started with JavaScript. I would love to know what you thought of my article, so please do leave me a comment, especially if there’s something you think could be improved.